Synchronous/Asynchronous & Blocking/Non-blocking
📚 Keywords 📚
- Synchronous
- Asynchronous
- Blocking
- Non-blocking
A term closely related to multitasking.
- Synchronous/Asynchronous : A perspective on whether or not tasks will be performed sequentially, paying attention to whether or not the requested tasks are completed.
- Blocking/Non-blocking : As the word suggests, it is a perspective on whether different tasks can be performed depending on whether the current task is blocked or not.
Although the difference in meaning between the two concepts is clear, they are often used interchangeably in programming.
- ex. JavaScript's
setTimeout()
function is generally called an asynchronous function, but it is also a non-blocking function. → The JavaScript asynchronous function that we call for convenience is actually an asynchronous + non-blocking function.
Synchronous / Asynchronous.
- Synchronous is interpreted to mean that work is executed according to time.
- Executing tasks sequentially means checking whether the requested tasks have been completed and processing them sequentially.
- Asynchronous is a form of negation with the prefix A in front.
- Contrary to motivation, since there is no consideration of whether the requested task has been completed or not, the next task is carried out as is.
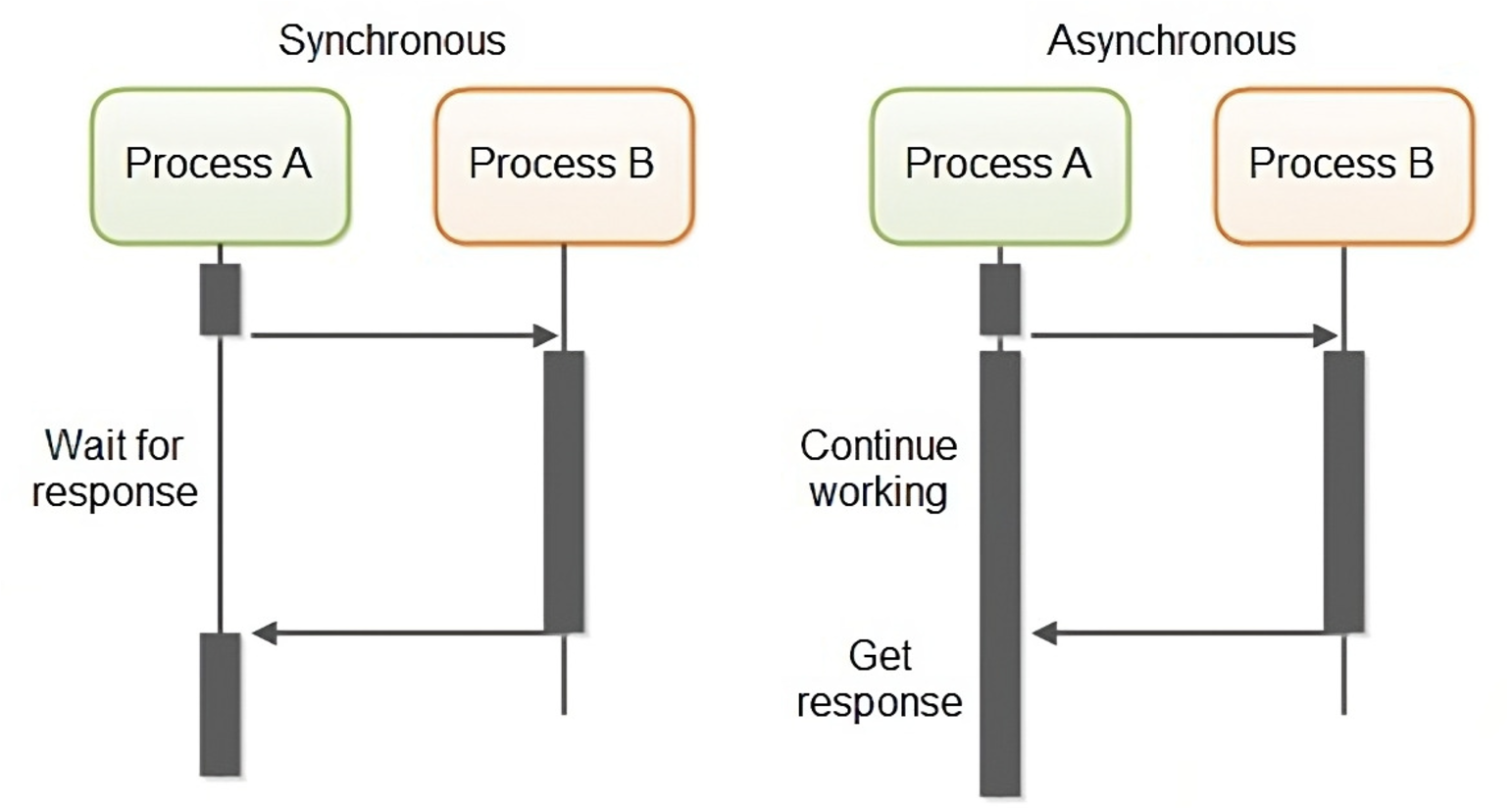
Performance benefits of Asynchronous.
- When slow tasks such as I/O tasks occur, you can perform multi-tasking by processing other tasks simultaneously without waiting.
- In the case of JavaScript, when a task is requested asynchronously, the task is authorized to the multi-threaded Web API built into the browser, and the main call stack and task are processed simultaneously. However, the JavaScript code execution itself is executed in the call stack, not the web API. Typical types of asynchronous tasks include animation execution, network communication, mouse and keyboard input, timers, etc.

Synchronous and Asynchronous differ in task order processing.
- The difference between synchronous and asynchronous is whether or not the requested task is completed. To put it simply, it can be seen as the difference in whether or not multiple requested tasks are processed sequentially.
- For example, let's assume there are three tasks, A, B, and C. If executed synchronously, it will be executed in the order A → B → C. If executed asynchronously, it will be executed in a random order such as A → C → B or C → A → B.
- To summarize, if three tasks are requested and the order is maintained in the response, it is synchronous. If you do not know which will come first, it can be considered asynchronous.
Blocking / Non-Blocking
- As the words imply, blocking and non-blocking are process execution methods that indicate whether or not to block (block, wait) the current task in order to process the work of another request.
- Blocking/non-blocking can be seen as whether or not the overall work flow itself is blocked. For example, when reading a file, if you read it in a blocking manner, you will wait until the file is finished reading, and if you read it in a non-blocking manner, you can do other work without having to read the entire file.

Difference between asynchronous and non-blocking concepts
JavaScript's setTimeout function is an asynchronous function and a non-blocking function. This is because each call is different depending on what perspective setTimeout is viewed from.
console.log("Start");
setTimeout(() => {
console.log("This will execute after 1 second!");
}, 1000);
console.log("End");
Start
End
This will execute after 1 second!
- When you run the above code, "Start" and "End" are output first, and after 1 second, "This will execute after 1 second!" is output. → The output order does not match the defined code line order.
- This is because the next console task was performed immediately after the
setTimeout
function without caring whether the timer task was completed. And the timer task completion alarm of thesetTimeout
function receives the value and outputs it through the callback function. Therefore,setTimeout
is asynchronous. - From another perspective, for the main function work, the
setTimeout
function was processed separately in the background without blocking the main function to perform its own timer work. Since the main function is not blocked, thesetTimeout
function is called and then the next console function is called. → Therefore,setTimeout
is non-blocking. - However, in the end, if you look only at the result you are pursuing, whether asynchronous or non-blocking, it can be seen as performing different tasks at the same time. Since this is a theoretical concept related to viewpoint, it is sometimes difficult to distinguish boundaries in actual code. So it seems that these are used interchangeably in programming. However, the exact meaning is different.
Asynchronous non-blocking and Callback Functions
- Asynchronous non-blocking refers to a method of executing in parallel without waiting for the results of other tasks. In this case, a callback function is used to determine whether other tasks are completed or to post-process the results.
- So are callback functions related to asynchronous? Does it have something to do with non-blocking? The answer is that both are related. Strictly speaking, a callback function is a technology that implements asynchronous non-blocking, not a concept.
- If you do not want to implement asynchronous non-blocking using a callback function because of callback hell, you can use the Promise object Visit Website or process it by emitting it as an event. There are many ways.
- The key point is that there are many technologies other than callbacks to implement asynchronous non-blocking, and callback is only one of them, and is not directly related to the concepts of synchronous/asynchronous & blocking/non-blocking.
/* Asynchronous + non-blocking server request operation implemented using callback function method */
$.ajax({
url: "https://jsonplaceholder.typicode.com/todos/1",
type: "GET",
dataType: "json",
success: function (data) {
// A callback function to be called if the request is successful.
console.log(data);
},
error: function (err) {
// A callback function to be called if the request fails.
throw err;
},
});
// You can perform other tasks at the same time you send the request.
console.log("Hello");
/* Asynchronous + non-blocking server request operation implemented using promise object method */
fetch("https://jsonplaceholder.typicode.com/todos/1")
.then((response) => {
return response.json();
})
.then((data) => {
console.log(data);
})
.catch((err) => {
throw err;
});
// You can perform other tasks at the same time you send the request.
console.log("Hello");
/* Asynchronous + non-blocking file download operation implemented using event method */
const fileReader = new FileReader(); // Create a FileReader object
const input = document.querySelector("input");
const file = input.files[0]; // Gets the file selected in the input tag
fileReader.addEventListener("load", function () {
console.log(fileReader.result); // When the file is finished loading, the contents are output.
});
fileReader.addEventListener("error", function (err) {
throw err;
});
// You can perform other tasks at the same time you send the request.
console.log("Hello");
Who has control?
- To more clearly distinguish between synchronous/asynchronous and blocking/non-blocking, the word 'control' is used.
- Simply put, control rights are the right to control the code of a function or the execution flow of a process.
- This concept is taken from the kernel of the operating system (OS) and is explained in I/O operations. → Blocking and Non-Blocking are distinguished by whether or not the called function (callee) immediately gives control to the called function (caller). If control is transferred, the thread will be blocked.